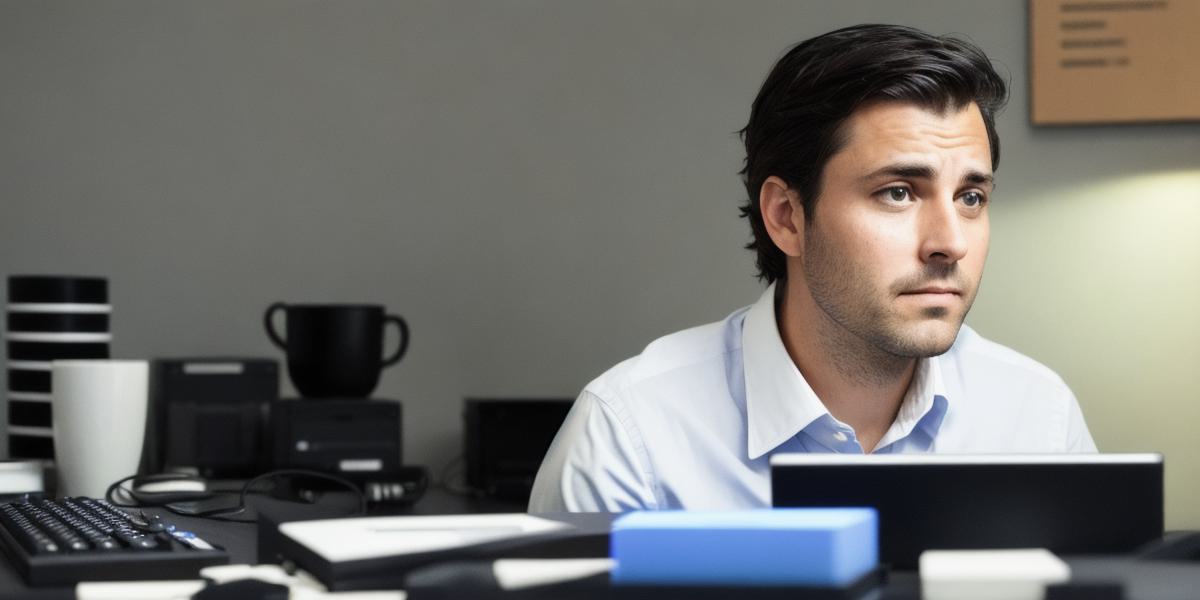
How to Fix Uncaught SyntaxError: Cannot use import statement outside a module
Are you getting frustrated with your code because of the "Uncaught SyntaxError: Cannot use import statement outside a module" error? This error can occur when you try to use the import statement in a file that is not part of a Node.js module. In this article, we will discuss how to fix this error and optimize your code for better performance.
First, let’s understand what a Node.js module is. A module is a self-contained unit of JavaScript code that can be imported into other files. Modules provide a way to organize your code into smaller, more manageable pieces. When you use an import statement in a file that is not part of a module, the browser throws an error because it cannot find the specified module.
To fix this error, you need to create a new module for the file that is throwing the error. To do this, simply add the "module.exports" object to the end of the file and export the necessary code.
For example:
const myModule (() > {
const foo 'bar';
return {
<h2> bar: foo,</h2>
};
})();
module.exports myModule;
Now that you have created a module, you can import it into other files using the "require" function.
For example:
const myModule require('./my-module');
console.log(myModule.bar); // Output: bar
By following these steps, you can fix the "Uncaught SyntaxError: Cannot use import statement outside a module" error and optimize your code for better performance. Remember to always use modules when working with JavaScript to keep your code organized and easy to maintain.